Output Devices Week
For the reminder, I explored Neopixel and speaker so that I can use it in the reminder band.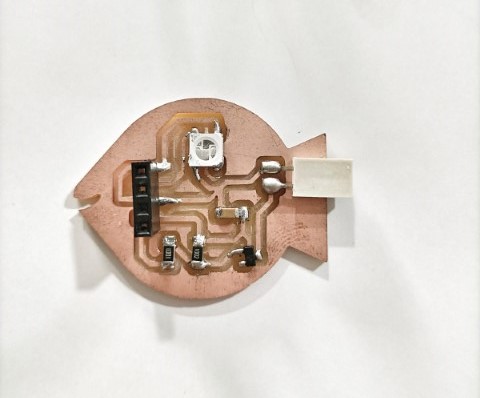
Neopixel as an Output device
NeoPixels are special LEDs - they're not just one light but three! Each NeoPixel contains tiny red, green, and blue lights all in one package.
To light up the Neopixel
#include < Adafruit_NeoPixel.h> #define LED_PIN 5 // Define the pin connected to the NeoPixel #define LED_COUNT 1 // Define the number of NeoPixels in the chain Adafruit_NeoPixel strip(LED_COUNT, LED_PIN, NEO_GRB + NEO_KHZ800); void setup() { // Initialize NeoPixel strip strip.begin(); strip.show(); // Initialize all pixels to 'off' } void loop() { // Set NeoPixel color to green strip.setPixelColor(0, 0, 255, 0); // Green color (R=0, G=255, B=0) strip.show(); // Update the NeoPixel strip with the new color }
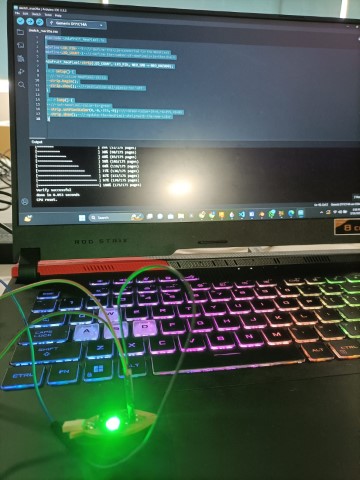
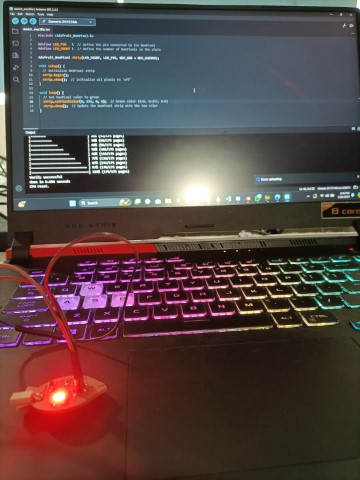
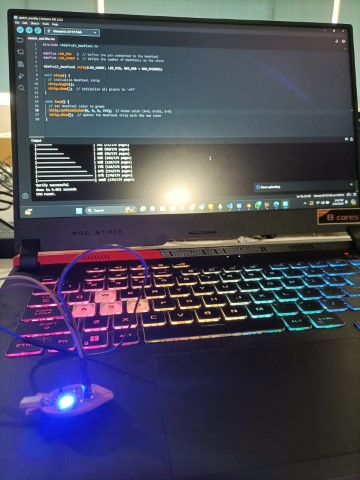
Changing this command, I got red colour. strip.setPixelColor(0, 255, 0, 0);
Changing this command again, I got blue colour. strip.setPixelColor(0, 0, 0, 255);
To colour cycle the neopixel
#include < Adafruit_NeoPixel.h> #define LED_PIN 5 // Define the pin connected to the NeoPixel #define LED_COUNT 1 // Define the number of NeoPixels in the chain Adafruit_NeoPixel strip(LED_COUNT, LED_PIN, NEO_GRB + NEO_KHZ800); void setup() { // Initialize NeoPixel strip strip.begin(); strip.show(); // Initialize all pixels to 'off' } void loop() { colorCycle(10); // Speed of color cycling (adjust as needed) } // Function to cycle through colors void colorCycle(uint8_t wait) { uint16_t i; for(i = 0; i < 256; i++) { // Set NeoPixel color strip.setPixelColor(0, Wheel((i) & 255)); strip.show(); delay(wait); // Wait between colors } } // Input a value 0 to 255 to get a color value // The colors are a transition r - g - b - back to r uint32_t Wheel(byte WheelPos) { if(WheelPos < 85) { return strip.Color(WheelPos * 3, 255 - WheelPos * 3, 0); } else if(WheelPos < 170) { WheelPos -= 85; return strip.Color(255 - WheelPos * 3, 0, WheelPos * 3); } else { WheelPos -= 170; return strip.Color(0, WheelPos * 3, 255 - WheelPos * 3); } }
Speaker as an output device
Since I was planning to use a speaker ,it is required to use a trasistor. I used MOSFET (Metal-Oxide-Semiconductor Field-Effect Transistor). It is a type of transistor commonly used in electronic circuits for switching and amplifying electronic signals. When using a MOSFET with a speaker, it's typically employed as a switch to control the flow of current through the speaker.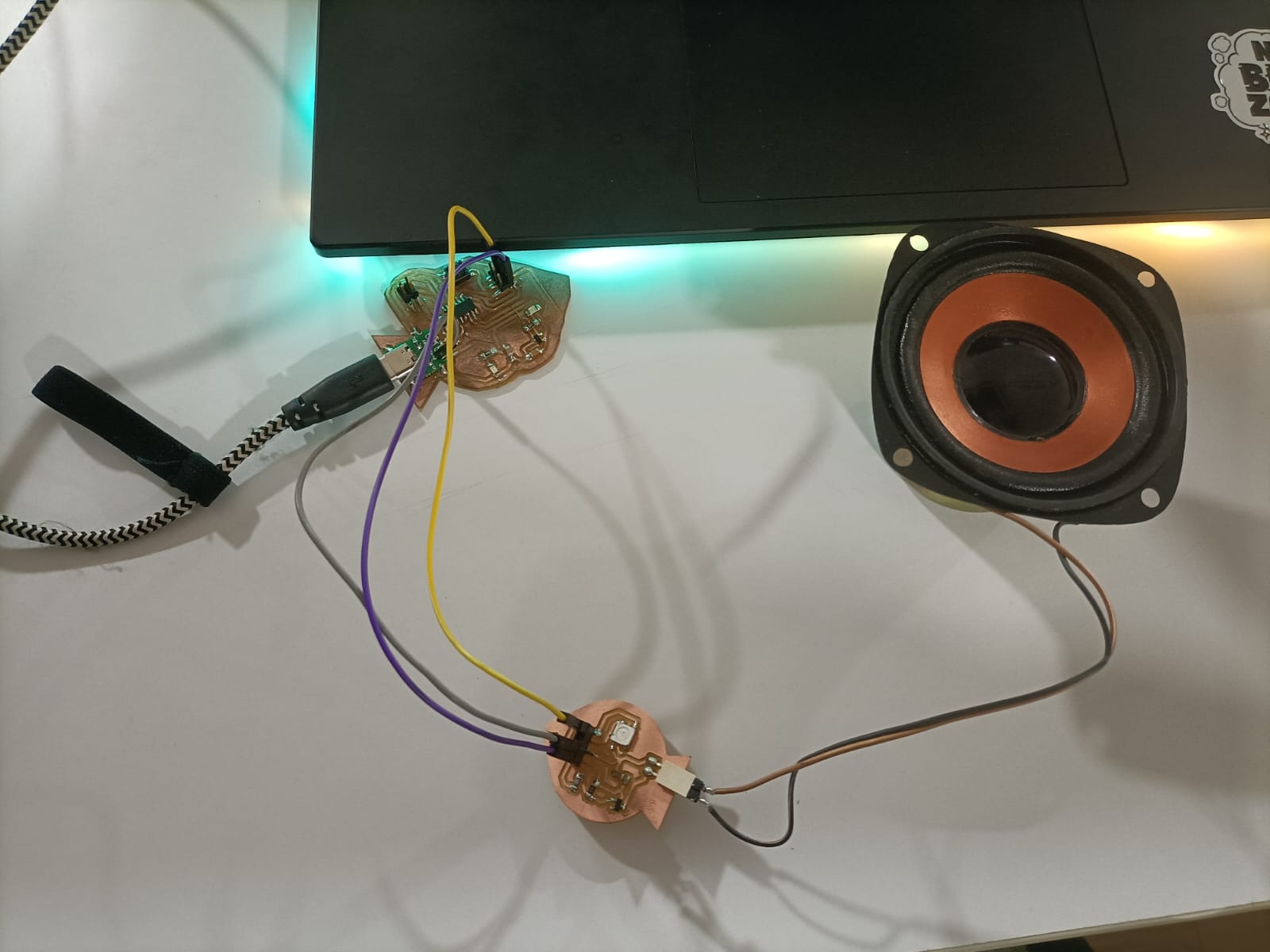
This code of Happy birthday is from Alfia's documentation. The link is here.
#define led 2 // led pin #define speakerPin 5 int length = 28; // the number of notes char notes[] = "GGAGcB GGAGdc GGxecBA yyecdc"; int beats[] = { 2, 2, 8, 8, 8, 16, 1, 2, 2, 8, 8, 8, 16, 1, 2, 2, 8, 8, 8, 8, 16, 1, 2, 2, 8, 8, 8, 16 }; int tempo = 150; void playTone(int tone, int duration) { for (long i = 0; i < duration * 1000L; i += tone * 2) { digitalWrite(speakerPin, HIGH); delayMicroseconds(tone); digitalWrite(speakerPin, LOW); delayMicroseconds(tone); } } void playNote(char note, int duration) { char names[] = { 'C', 'D', 'E', 'F', 'G', 'A', 'B', 'c', 'd', 'e', 'f', 'g', 'a', 'b', 'x', 'y' }; int tones[] = { 1915, 1700, 1519, 1432, 1275, 1136, 1014, 956, 834, 765, 593, 468, 346, 224, 655, 715 }; int SPEE = 5; // play the tone corresponding to the note name for (int i = 0; i < 17; i++) { if (names[i] == note) { int newduration = duration / SPEE; playTone(tones[i], newduration); } } } void setup() { pinMode(speakerPin, OUTPUT); //buzzer pinMode(led, OUTPUT); digitalWrite(led, HIGH); delay(1000); digitalWrite(led, LOW); } void loop() { for (int i = 0; i < length; i++) { if (notes[i] == ' ') { delay(beats[i] * tempo); // rest } else { playNote(notes[i], beats[i] * tempo); } // pause between notes delay(tempo); } delay(100); }